Vue3 可以使用 v-for 指令來渲染一個基於來源數組的列表做一些動態綁定的應用
基本用法
- 利用 ref 宣告一個 items – json 物件
- 在 tamplate 中 透過 v-for 將 其值渲染出來
- v-for 用法 等同於 foreach 用法 “item in items” 這邊的 item 是宣告出來的物件
- 這邊可以省略 index 改成 指宣告 item in items上面說的用法
<script setup>
import { ref } from 'vue'
const items = ref([
{title: "home"},
{title: "detail"},
{title: "setting"},
{title: "advance"}
]
)
</script>
<template>
<ul>
<li v-for="(item, index) in items">
{{index + "-"+ item.title }}
</li>
</ul>
</template>

reactive – key, value 用法
<script setup>
import { ref, reactive } from 'vue'
const myObject = reactive({
title: 'Rororo Vue Web',
author: 'Rororo',
publishedAt: '20240331'
})
</script>
<template>
<ul>
<li v-for="value in myObject">
{{ value }}
</li>
</ul>
</template>
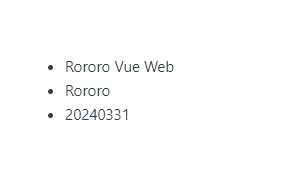
進階應用
- v-for 配合 ref 變數 有一個好處就是可以動態綁定與動態修改頁面上的值 , 與傳統的jquery 方法 差很多
- 我們這邊參考別人的範例: https://cn.vuejs.org/tutorial/#step-7
- 新增 資料 可以 快速出現在 li 清單中
- 刪除也可以快速的消失在 li 清單中
- 不需額外撰寫刪除與新增的前端頁面語法 ,只需要專注在 value 就好
<script setup>
import { ref } from 'vue'
let id = 0
const newTodo = ref('')
const todos = ref([
{ id: id++, text: 'Learn HTML' },
{ id: id++, text: 'Learn JavaScript' },
{ id: id++, text: 'Learn Vue' }
])
function addTodo() {
todos.value.push({ id: id++, text: newTodo.value })
newTodo.value = ''
}
function removeTodo(todo) {
todos.value = todos.value.filter((t) => t !== todo)
}
</script>
<template>
<form @submit.prevent="addTodo">
<input v-model="newTodo" required placeholder="new todo">
<button>Add Todo</button>
</form>
<ul>
<li v-for="todo in todos" :key="todo.id">
{{ todo.text }}
<button @click="removeTodo(todo)">X</button>
</li>
</ul>
</template>
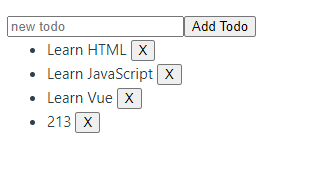